TypeScript读取SQLite数据库
在TypeScript中读取SQLite数据库中的SVG源数据,并在HTML中展示,可以通过以下几个步骤实现:
-
安装依赖项:需要安装sqlite3
和相关的TypeScript类型定义文件。
-
建立SQLite数据库连接:使用sqlite3
模块连接到SQLite数据库并执行查询以读取SVG数据。
-
设置一个Express服务器:使用Express来设置一个简单的服务器以处理数据的读取和返回。
-
前端展示:通过HTML和JavaScript将从服务器获取的SVG数据嵌入到网页中进行展示。
下面是一个完整的示例:
第一步:安装依赖项
使用npm安装必要的依赖项:
| npm install express sqlite3 @types/express @types/sqlite3
|
第二步:建立SQLite数据库连接并读取SVG数据
创建一个文件server.ts
,用于设置服务器和读取数据库数据:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32 | import express from 'express';
import sqlite3 from 'sqlite3';
import { open } from 'sqlite';
const app = express();
const port = 3000;
// 打开SQLite数据库
async function openDb() {
return open({
filename: './database.sqlite',
driver: sqlite3.Database
});
}
// 设置一个GET路由以返回SVG数据
app.get('/svg/:id', async (req, res) => {
const id = req.params.id;
const db = await openDb();
const result = await db.get('SELECT svg_data FROM svgs WHERE id = ?', [id]);
if (result) {
res.send(result.svg_data);
} else {
res.status(404).send('SVG not found');
}
await db.close();
});
// 启动服务器
app.listen(port, () => {
console.log(`Server is running at http://localhost:${port}`);
});
|
第三步:设置前端页面展示SVG
创建一个文件index.html
,用于展示SVG数据:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29 | <!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Display SVG</title>
</head>
<body>
<div id="svg-container"></div>
<script>
async function fetchAndDisplaySvg(id) {
try {
const response = await fetch(`/svg/${id}`);
if (!response.ok) {
throw new Error('SVG not found');
}
const svgData = await response.text();
document.getElementById('svg-container').innerHTML = svgData;
} catch (error) {
console.error('Error fetching SVG:', error);
}
}
// Fetch and display SVG with ID 1 as an example
fetchAndDisplaySvg(1);
</script>
</body>
</html>
|
第四步:编译并运行
-
编译TypeScript文件:
-
启动服务器:
-
打开浏览器: 访问http://localhost:3000
,应该能看到数据库中存储的SVG被正确加载和展示。
数据库初始化(可选)
如果还没有创建数据库和表,可以通过以下步骤来初始化:
- 创建一个文件
init-db.ts
,内容如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18 | import sqlite3 from 'sqlite3';
import { open } from 'sqlite';
async function openDb() {
return open({
filename: './database.sqlite',
driver: sqlite3.Database
});
}
async function setup() {
const db = await openDb();
await db.exec('CREATE TABLE IF NOT EXISTS svgs (id INTEGER PRIMARY KEY, svg_data TEXT)');
await db.run('INSERT INTO svgs (svg_data) VALUES (?)', [`<svg xmlns="http://www.w3.org/2000/svg" width="100" height="100"><circle cx="50" cy="50" r="40" stroke="black" stroke-width="3" fill="red" /></svg>`]);
await db.close();
}
setup();
|
- 运行初始化脚本:
| tsc init-db.ts
node init-db.js
|
这样,数据库和表会被创建,并插入一个示例SVG数据。
通过以上步骤,你可以实现从SQLite数据库中读取SVG数据并在HTML页面中展示。
捐赠本站(Donate)
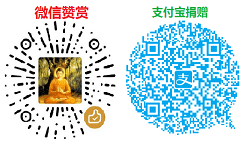
如您感觉文章有用,可扫码捐赠本站!(If the article useful, you can scan the QR code to donate))